使用 Spring 的 IOC 实现账户的 CRUD (XML配置)
需求
实现账户的 CRUD 操作
开始编写
导入maven依赖(pom.xml)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.12.RELEASE</version>
</dependency>
<dependency>
<groupId>commons-dbutils</groupId>
<artifactId>commons-dbutils</artifactId>
<version>1.6</version>
</dependency>
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.1</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.16</version>
</dependency>创建数据库
1
2
3
4
5
6
7
8
9
10
11DROP TABLE IF EXISTS `account`;
CREATE TABLE `account` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(40) DEFAULT NULL,
`money` float DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
INSERT INTO `account` VALUES ('1', 'aaa', '1000');
INSERT INTO `account` VALUES ('2', 'bbb', '1000');
INSERT INTO `account` VALUES ('3', 'ccc', '1000');编写实体类(
cn.imzjw.entity.Account.java
)1
2
3
4
5
6
7
8
9
public class Account implements Serializable {
private Integer id;
private String name;
private float money;
}编写持久层代码(
cn.imzjw.dao.IAccountDao.java
)1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31public interface IAccountDao {
/**
* 查询所有
*
* @return
*/
List<Account> findAllAccount();
/**
* 查询一个
*
* @return
*/
Account findAccountById(Integer id);
/**
* 保存账户
*/
void saveAccount(Account account);
/**
* 删除账户
*/
void delAccount(Integer id);
/**
* 更新账户
*/
void updateAccount(Account account);
}持久层接口实现类(
cn.imzjw.dao.impl.AccountDaoImpl
)1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59public class AccountDaoImpl implements IAccountDao {
private QueryRunner queryRunner;
public void setQueryRunner(QueryRunner queryRunner) {
this.queryRunner = queryRunner;
}
public List<Account> findAllAccount() {
try {
return queryRunner.query("SELECT * FROM account",
new BeanListHandler<Account>(Account.class));
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
public Account findAccountById(Integer id) {
try {
return queryRunner.query("SELECT * FROM account WHERE id = ?",
new BeanHandler<>(Account.class), id);
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
public void saveAccount(Account account) {
try {
queryRunner.update("INSERT INTO account(name, money) VALUES(?, ?)",
account.getName(), account.getMoney());
} catch (SQLException e) {
e.printStackTrace();
}
}
public void delAccount(Integer id) {
try {
queryRunner.update("DELETE FROM account WHERE id = ?", id);
} catch (SQLException e) {
e.printStackTrace();
}
}
public void updateAccount(Account account) {
try {
queryRunner.update("UPDATE account SET name = ?, money = ? WHERE id = ?",
account.getName(), account.getMoney(), account.getId());
} catch (SQLException e) {
e.printStackTrace();
}
}
}编写业务层代码(
cn.imzjw.service.IAccountService.java
)1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31public interface IAccountService {
/**
* 查询所有
*
* @return
*/
List<Account> findAllAccount();
/**
* 查询一个
*
* @return
*/
Account findAccountById(Integer id);
/**
* 保存账户
*/
void saveAccount(Account account);
/**
* 删除账户
*/
void delAccount(Integer id);
/**
* 更新账户
*/
void updateAccount(Account account);
}编写业务层接口实现类(
cn.imzjw.service.impl.AccountServiceImpl.java
)1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33public class AccountServiceImpl implements IAccountService {
private IAccountDao accountDao;
public void setAccountDao(IAccountDao accountDao) {
this.accountDao = accountDao;
}
public List<Account> findAllAccount() {
return accountDao.findAllAccount();
}
public Account findAccountById(Integer id) {
return accountDao.findAccountById(id);
}
public void saveAccount(Account account) {
accountDao.saveAccount(account);
}
public void delAccount(Integer id) {
accountDao.delAccount(id);
}
public void updateAccount(Account account) {
accountDao.updateAccount(account);
}
}在
resources
下new
一个xml
配置文件,命名随意,但不可中文,配置文件代码如下:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--配置 service -->
<bean id="accountService" class="cn.imzjw.service.impl.AccountServiceImpl">
<!--注入 accountDao -->
<property name="accountDao" ref="accountDaoImpl"/>
</bean>
<!--配置持久层-->
<bean id="accountDaoImpl" class="cn.imzjw.dao.impl.AccountDaoImpl">
<!--注入 queryRunner -->
<property name="queryRunner" ref="queryRunner"/>
</bean>
<!--配置 queryRunner-->
<bean id="queryRunner" class="org.apache.commons.dbutils.QueryRunner" scope="singleton">
<!--注入数据源-->
<constructor-arg name="ds" ref="dataSource"/>
</bean>
<!--配置数据源-->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<!--连接数据库的必备信息-->
<property name="driverClass" value="com.mysql.jdbc.Driver"/>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/spring"/>
<property name="user" value="garvey"/>
<property name="password" value="garvey"/>
</bean>
</beans>
经过如上一番配置,现在开始进行测试
在test
包下新建测试类,如图,这是我的项目结构
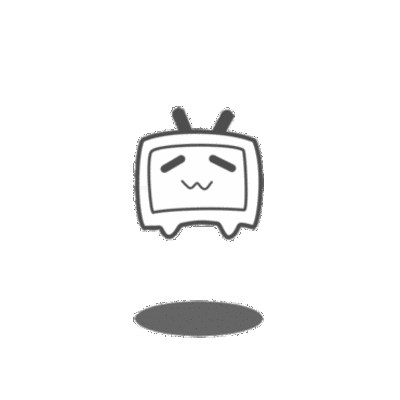
测试
编写测试类代码(cn.imzjw.test.AccountServiceTest.java
)
1 | public class AccountServiceTest { |
查询所有运行结果为:
1 | Account(id=1, name=aaa, money=1000.0) |
查询一个运行结果为:
1 | Account(id=1, name=aaa, money=1000.0) |
保存账户运行结果为:
1 | Account(id=1, name=aaa, money=1000.0) |
更新账户运行结果为:
1 | Account(id=1, name=aaa, money=1000.0) |
删除账户运行结果为:
1 | Account(id=1, name=aaa, money=1000.0) |
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 小嘉的部落格!
评论