前言
曾经我们部署 Spring Boot 项目的时候,还要手动 mvn clean install,然后再把 JAR 包上传到服务器,最后 java -jar
,后面有了 jenkins CI 工具让我们一劳永逸,但是本文讲的不是 jenkins,而是 GitHub Actions
GitHub Actions
GitHub Actions 是 GitHub 推出的持续集成(Continuous integration 简称 CI)服务,它提供了配置非常不错的虚拟服务器环境,基于它可以进行构建、测试、打包、部署项目。简单来讲就是将软件开发中的一些流程交给云服务器自动化处理,比如我们开发者吧代码 push
到 GitHub 后它就会自动测试、编译、发布,有了这么一个持续集成服务之后开发者就可以专心于写代码,其他什么琐事就不用管了,大大的提高开发效率。
在原先 2019 年 11 月的时候,GitHub Actions 还处在测试阶段,需要申请才能使用,而现在已经完全可以免费使用了,免费归免费,但是 GitHub 官方也对使用 GitHub Actions 的做出了一些限制。 使用限制
正式开始
环境
- Spring Boot 2.2.6.RELEASE
- IntelliJ IDEA 2020.3.4
- JDK 1.8
- Apache Maven 3.6.3
- 腾讯云服务器(CentOS 7.6)
首先创建一个 Spring Boot 工程,勾选 Web 依赖
完整 pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.6.RELEASE</version> <relativePath/> </parent> <groupId>cn.imzjw.workflow.github</groupId> <artifactId>github-actions-springboot</artifactId> <version>0.0.1-SNAPSHOT</version> <name>github-actions-springboot</name> <description>Demo project for Spring Boot</description> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <maven-surefire-plugin.version>2.19.1</maven-surefire-plugin.version> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>2.2.6.RELEASE</version> <executions> <execution> <goals> <goal>build-info</goal> <goal>repackage</goal> </goals> </execution> </executions> </plugin>
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> </plugin>
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>${maven-surefire-plugin.version}</version> </plugin>
<plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>cobertura-maven-plugin</artifactId> <version>2.7</version> <configuration> <formats> <format>html</format> <format>xml</format> </formats> <check/> </configuration> </plugin>
</plugins> </build> </project>
|
新建一个类,用来测试
1 2 3 4 5 6 7 8
| @RestController public class ActionsController {
@GetMapping("actions") public String actions() { return "使用 GitHub Actions 部署至服务器"; } }
|
代码就这么点,然后就进行编写 actions 代码,在你项目的根目录下创建 .github/workflows/main.yml
除了那俩文件夹名固定的,main.yml
文件名可以随便乱取。
我的项目结构
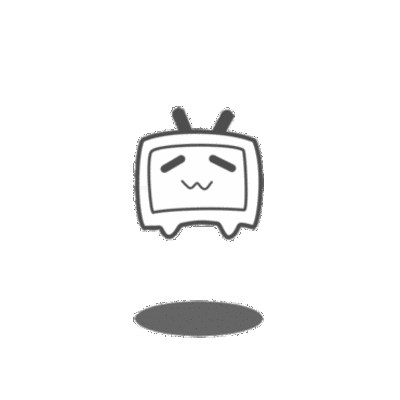
编辑 main.yml 文件,添加如下配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| name: Java CI with Maven
on: push: branches: [ master ] pull_request: branches: [ master ]
jobs: compile: runs-on: ubuntu-latest name: Running Java ${{ matrix.java }} compile steps: - uses: actions/checkout@v2 - name: Set up JDK 1.8 uses: actions/setup-java@v1 with: java-version: 1.8 - name: 缓存 Maven 依赖 uses: actions/cache@v2 with: path: ~/.m2/repository key: ${{ runner.os }}-maven-${{ hashFiles('**/pom.xml') }} restore-keys: | ${{ runner.os }}-maven- - name: 编译代码 run: mvn compile - name: Deploy the JAR file to the remote server uses: actions/checkout@v2 - name: Set up JDK 1.8 uses: actions/setup-java@v1 with: java-version: 1.8 - name: Generate the package run: mvn -B package --file pom.xml -Dmaven.test.skip=true - name: 将 JAR 包部署到服务器 uses: garygrossgarten/github-action-scp@release with: local: target/github-actions-springboot-0.0.1-SNAPSHOT.jar remote: blog.imzjw.cn/actions/github-actions-springboot.jar host: ${{ secrets.HOST }} username: ${{ secrets.SSH_USER }} password: ${{ secrets.SSH_PASSWORD }} - name: 在服务器上执行 java -jar,请确保服务器安装了 JDK if: always() uses: fifsky/ssh-action@master with: command: | cd blog.imzjw.cn/actions/ && java -jar github-actions-springboot.jar & host: ${{ secrets.HOST }} user: ${{ secrets.SSH_USER }} pass: ${{ secrets.SSH_PASSWORD }} args: "-tt"
|
随后到 GitHub 创建一个新的存储库 ,在仓库依次点击 Setting -> Secrets -> New repository secret
三个密钥都必填 *
Name | Value |
---|
HOST | 填你的服务器 IP |
SSH_USER | 服务器用户名 |
SSH_PASSWORD | 服务器密码 |
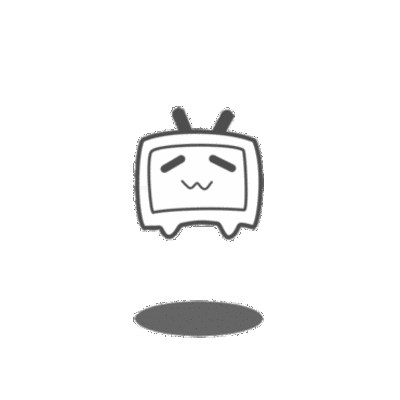
在这之前先检查一下服务器是否已安装 JDK,否则 java -jar
会失败
jdk-8u181-linux-x64.tar.gz
下载地址
安装就不用说了…直接 tar 解压, vim /etc/profile 添加 JDK 路径, source /etc/profile 生效
最后直接 push 项目到 GitHub 存储仓中
点击仓库中的 Actions 可以看到已经跑起来了,点进去,可以看到每个步骤的工作流程!
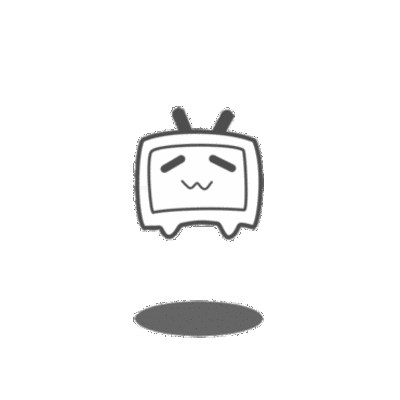
设置的密钥都是安全加密的,duck 不必担心。
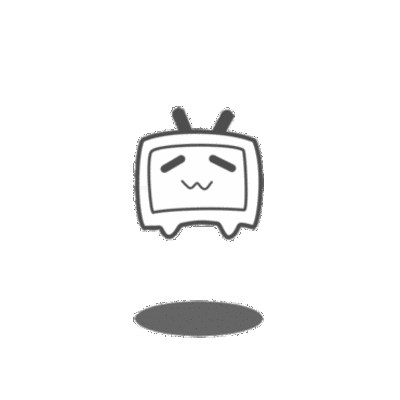
等待 Actions 工作完成之后,用 SSH 工具连接到你的服务器,执行
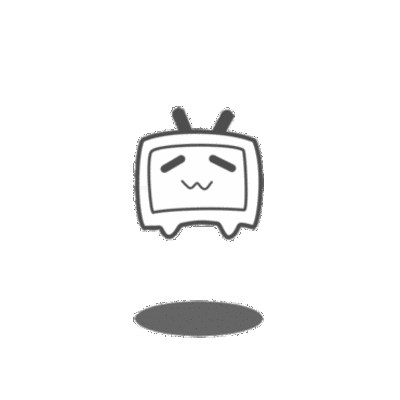
完美!
最后浏览器访问 http://IP:8080/actions
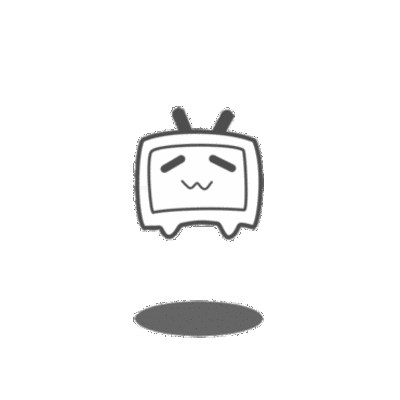
参考:
感谢:
本文 GitHub 仓库地址
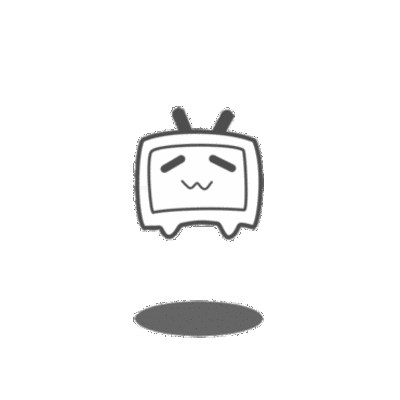
结语
我希望你喜欢这篇文章并学会了如何使用 GitHub Actions 来自动化(CI / CD Workflow)部署项目!