虽然 ssm 整合教程都烂大街了,而我这篇文章也仅仅是我今天做为复习记录一下… 怎么说也踩到坑了(真的太久没配置了),但是我不会告诉你的,总而言之
spring boot 真香
环境要求
- IDEA(2018.3)
- MySQL 5.7
- Tomcat 9.0.31
- Maven 3.6.3
数据库环境
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| DROP TABLE IF EXISTS `book`; CREATE TABLE `book` ( `book_id` int(10) NOT NULL AUTO_INCREMENT COMMENT '书id', `book_name` varchar(100) NOT NULL COMMENT '书名', `book_counts` int(11) NOT NULL COMMENT '数量', `detail` varchar(200) NOT NULL COMMENT '描述', KEY `book_id` (`book_id`) ) ENGINE=InnoDB AUTO_INCREMENT=25 DEFAULT CHARSET=utf8mb4;
INSERT INTO `book` VALUES ('1', 'Java', '1', '从入门到放弃'); INSERT INTO `book` VALUES ('2', 'MySQL', '10', '从删库到跑路');
|
基本环境搭建
新建 Maven Web 项目(注意选择,选择没有 22 的 webapp
)
导入依赖(pom.xml)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93
| <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.11</version> <scope>test</scope> </dependency>
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.3.2</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>5.3.2</version> </dependency>
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.47</version> </dependency>
<dependency> <groupId>com.mchange</groupId> <artifactId>c3p0</artifactId> <version>0.9.5.2</version> </dependency>
<dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency>
<dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.6</version> </dependency> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis-spring</artifactId> <version>2.0.6</version> </dependency>
<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.16</version> </dependency>
</dependencies>
<build> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> <resource> <directory>src/main/resources</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>false</filtering> </resource> </resources> </build>
|
编写 web.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| <?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" version="4.0">
<filter> <filter-name>encoding</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>utf-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>encoding</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
<servlet> <servlet-name>springmvc</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:spring/spring-web.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet>
<servlet-mapping> <servlet-name>springmvc</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
<listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:spring/spring-*.xml</param-value> </context-param>
</web-app>
|
在 resources
下新建 db.properties
并加入以下代码
1 2 3 4
| jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/ssm?useUnicode=true&characterEncoding=utf-8&serverTimezone=UTC jdbc.username=root jdbc.password=root
|
在 resources
下新建 mybatis 配置文件(mybatis-config.xml)名字随意,但为了规范
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration>
<settings> <setting name="useGeneratedKeys" value="true"/> <setting name="mapUnderscoreToCamelCase" value="true"/> </settings>
</configuration>
|
在 resources
下新建 spring
文件夹
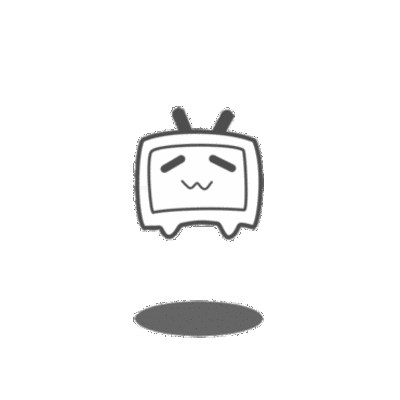
接着在 spring
文件夹下新建 spring-dao.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:db.properties"/>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <property name="driverClass" value="${jdbc.driver}"/> <property name="jdbcUrl" value="${jdbc.url}"/> <property name="user" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> <property name="maxPoolSize" value="30"/> <property name="minPoolSize" value="10"/> <property name="autoCommitOnClose" value="false"/> <property name="checkoutTimeout" value="10000"/> <property name="acquireRetryAttempts" value="2"/> </bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource"/> <property name="mapperLocations" value="classpath:mapper/*Mapper.xml"/> <property name="configLocation" value="classpath:mybatis-config.xml"/> <property name="typeAliasesPackage" value="cn.imzjw.ssm.entity"/> </bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="cn.imzjw.ssm.mapper"/> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/> </bean>
</beans>
|
编写 spring-service.xml
(resources / spring / spring-service.xml)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd">
<import resource="spring-dao.xml"/>
<context:component-scan base-package="cn.imzjw.ssm.service"/>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"/> </bean>
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
|
spring-web.xml
(resources / spring / spring-web.xml)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation=" http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<mvc:annotation-driven/>
<context:component-scan base-package="cn.imzjw.ssm.controller"> <context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/> </context:component-scan>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/jsp/"/> <property name="suffix" value=".jsp"/> </bean>
</beans>
|
接着在 resources 下新建 mapper 文件夹,然后不用管他先,如此如此基本的环境就已经搭建好了
这是我的项目结构
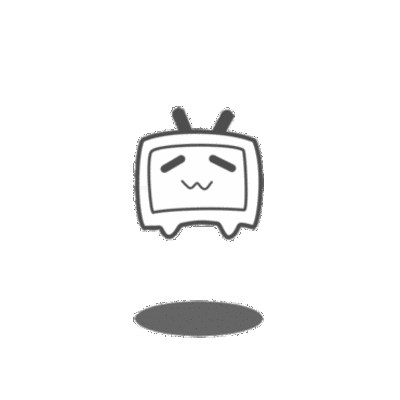
现在开始上代码啦
实体类 Book
(cn.imzjw.ssm.entity.Book.java)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
@Data @NoArgsConstructor @AllArgsConstructor public class Book {
private int bookId;
private String bookName;
private int bookCounts;
private String detail; }
|
mapper
层(cn.imzjw.ssm.mapper.BookMapper.java)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
|
public interface BookMapper {
int addBook(Book book);
int deleteBookById(int bookId);
int updateBook(Book book);
Book selectBookById(int bookId);
List<Book> selectAllBook();
Book selectBookName(String bookName); }
|
编写接口对应的 Mapper.xml,在 resources / mapper / 新建 BookMapper.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="cn.imzjw.ssm.mapper.BookMapper">
<insert id="addBook" parameterType="Book"> INSERT INTO book (book_name, book_counts, detail) VALUES (#{bookName}, #{bookCounts}, #{detail}) </insert>
<delete id="deleteBookById" parameterType="int"> DELETE FROM book WHERE book_id = #{bookId} </delete>
<update id="updateBook" parameterType="Book"> UPDATE book SET book_name = #{bookName}, book_counts = #{bookCounts}, detail = #{detail} WHERE book_id = #{bookId} </update>
<select id="selectBookById" resultType="Book"> SELECT * FROM book WHERE book_id = #{book_id} </select>
<select id="selectAllBook" resultType="Book"> SELECT * FROM book </select>
<select id="selectBookName" resultType="Book"> SELECT * FROM book WHERE bookName = #{bookName} </select>
</mapper>
|
service
层(cn.imzjw.ssm.service.BookService.java)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| public interface BookService {
int addBook(Book book);
int deleteBookById(int bookId);
int updateBook(Book book);
Book selectBookById(int bookId);
List<Book> selectAllBook();
Book selectBookName(String bookName); }
|
service
层实现类(cn.imzjw.ssm.service.impl.BookServiceImpl.java)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
|
@Service public class BookServiceImpl implements BookService {
@Autowired private BookMapper bookMapper;
@Override public int addBook(Book book) { return bookMapper.addBook(book); }
@Override public int deleteBookById(int bookId) { return bookMapper.deleteBookById(bookId); }
@Override public int updateBook(Book book) { return bookMapper.updateBook(book); }
@Override public Book selectBookById(int bookId) { return bookMapper.selectBookById(bookId); }
@Override public List<Book> selectAllBook() { return bookMapper.selectAllBook(); }
@Override public Book selectBookName(String bookName) { return bookMapper.selectBookName(bookName); } }
|
controller
层(cn.imzjw.ssm.controller.BookController.java)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110
|
@Controller public class BookController {
@Autowired private BookService bookService;
@RequestMapping("/") public String index() { return "redirect:listBook"; }
@RequestMapping("listBook") public String list(Model model) { model.addAttribute("list", bookService.selectAllBook()); return "allBook"; }
@RequestMapping("/toAddBook") public String toAddPaper() { return "addBook"; }
@PostMapping("/addBook") public String addBook(Book book) { bookService.addBook(book); return "redirect:listBook"; }
@RequestMapping("/toUpdate") public String toUpdatePaper(int id, Model model) { model.addAttribute("toBook", bookService.selectBookById(id)); return "updateBook"; }
@PostMapping("/updateBook") public String updateBook(Book book) { bookService.updateBook(book); return "redirect:listBook"; }
@RequestMapping("delBook") public String delBook(int id) { bookService.deleteBookById(id); return "redirect:listBook"; }
@RequestMapping("/selectBook") public String selectBook(String selectBookName, Model model) { Book book = bookService.selectBookName(selectBookName); List<Book> list = new ArrayList<>(); list.add(book); if (book == null) { model.addAttribute("error", "*未查询到相关数据"); } model.addAttribute("list", list); return "allBook"; } }
|
接着在 WEB-INF
下新建 jsp 文件夹
,然后在 jsp
文件夹新建 allBook.jsp
(书籍展示页面)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78
| <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>书籍展示</title> <%--引入BootStrap--%> <link rel="stylesheet" href="https://npm.elemecdn.com/bootstrap@3.3.7/dist/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> </head> <body>
<div class="container"> <div class="row clearfix"> <div class="col-md-12 column"> <div class="page-header text-center"> <h1> <small>书籍管理系统</small> </h1> </div> </div> </div>
<div class="row"> <div class="col-md-4 column"> <a class="btn btn-default" href="${pageContext.request.contextPath}/listBook">显示全部</a> <a class="btn btn-default" href="${pageContext.request.contextPath}/toAddBook">新增书籍</a> </div>
<form action="${pageContext.request.contextPath}/selectBook" method="post"> <div class="input-group col-md-3" style="float: right"> <input type="text" class="form-control" name="selectBookName" placeholder="请输入要查询的书籍"> <span class="input-group-btn"> <button class="btn btn-success btn-search">搜索</button> </span> </div> </form> </div> <br> <div class="row clearfix"> <div class="col-md-12 column"> <table class="table table-hover table-striped"> <thead> <th>书籍编号</th> <th>书籍名称</th> <th>书籍数量</th> <th>书籍描述</th> <th>操作</th> </thead> <tbody> <c:forEach var="book" items="${list}"> <tr> <td>${book.bookId}</td> <td>${book.bookName}</td> <td>${book.bookCounts}</td> <td>${book.detail}</td> <td> <a href="${pageContext.request.contextPath}/toUpdate?id=${book.bookId}">修改</a> | <a href="${pageContext.request.contextPath}/delBook?id=${book.bookId}">删除</a> </td> </tr> </c:forEach> </tbody> </table> </div> </div> <div class="row clearfix"> <div class="col-md-12 column"> <div class=" text-right"> <h3> <small>${error}</small> </h3> </div> </div> </div> </div> </body> </html>
|
添加书籍页面(WEB-INF / jsp / addBook.jsp
)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>新增书籍</title> <%--引入BootStrap--%> <link rel="stylesheet" href="https://npm.elemecdn.com/bootstrap@3.3.7/dist/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> </head> <body>
<div class="container"> <div class="row clearfix"> <div class="col-md-12 column"> <div class="page-header text-center"> <h1> <small>新增书籍</small> </h1> </div> </div> </div>
<form action="${pageContext.request.contextPath}/addBook" method="post" class="form-inline text-center"> <div class="form-group"> <label>书籍名称:</label> <input type="text" name="bookName" class="form-control" required> </div>
<div class="form-group"> <label>书籍数量:</label> <input type="text" name="bookCounts" class="form-control" required> </div>
<div class="form-group"> <label>书籍描述:</label> <input type="text" name="detail" class="form-control" required> </div> <button type="submit" class="btn btn-info">点击添加</button> </form> </div> </body> </html>
|
修改书籍页面(WEB-INF / jsp / updateBook.jsp
)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>修改书籍</title> <%--引入BootStrap--%> <link rel="stylesheet" href="https://npm.elemecdn.com/bootstrap@3.3.7/dist/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> </head> <body>
<div class="container">
<div class="row clearfix"> <div class="col-md-12 column"> <div class="page-header text-center"> <h1> <small>修改书籍</small> </h1> </div> </div> </div>
<form action="${pageContext.request.contextPath}/updateBook" method="post" class="form-inline text-center"> <%--隐藏域--%> <input type="hidden" name="bookId" value="${toBook.bookId}">
<div class="form-group"> <label>书籍名称:</label> <input type="text" name="bookName" class="form-control" value="${toBook.bookName}" required> </div>
<div class="form-group"> <label>书籍数量:</label> <input type="text" name="bookCounts" class="form-control" value="${toBook.bookCounts}" onkeyup="this.value=this.value.replace(/\D/g,'')" onafterpaste="this.value=this.value.replace(/\D/g,'')" required> </div>
<div class="form-group"> <label>书籍描述:</label> <input type="text" name="detail" class="form-control" value="${toBook.detail}" required> </div>
<button type="submit" class="btn btn-default">点击修改</button> </form> </div> </body> </html>
|
配置好 Tomcat
直接启动访问 http://localhost:8080
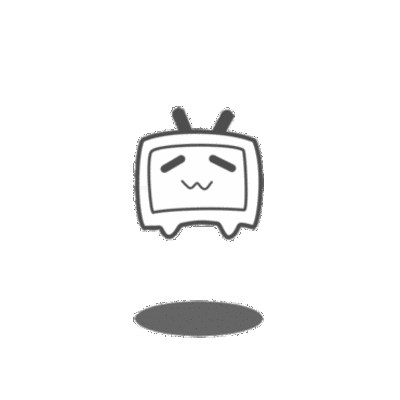